Introduction I²C (built-in integrated circuit) is a 2-wire interface that enables bidirectional communication between integrated circuits. This application note introduces the maxqi2c library, which is a software I²C driver for the MAXQ2000 microcontroller (µC).
The maxqi2c library is written in extended C language and compiled by MAXQ's IAR embedded platform. It consists of two files: maxqi2c.h and maxqi2c.c. When these files are included in the MAXQ2000 firmware project, any GPIO pin of µC can be used to achieve 100kHz or 400kHz flexible I²C communication.
The MAXQ series of microcontrollers have high-speed, flexible GPIO modules and independent I / O power supply, suitable for applications such as bit splitting.
The example project files discussed in this application note can be downloaded from the Maxim Integrated Products website. Users configuring the maxqi2c library should copy the maxqi2c library files (maxqi2c.h and maxqi2c.c) to the MAXQ2000 project directory, configure the files, and establish the required I2C interface. All the configuration is achieved by editing the following code (Listing 1), which is located at the beginning of the maxqi2c.h source file:
Listing 1. maxi2c.h user-defined code. / * USER MUST CUSTOMIZE THE FOLLOWING DEFINE STMTS-START * / // Enter the port used for SDA and SCL #define SDA_PORT 0 #define SCL_PORT 0 // Enter the pin used for SDA and SCL #define SDA_PORT_BIT 0 #define SCL_PORT_BIT 1 / / Uncomment one of these define statements to select I2C bus speed #define I2C_400_KHZ // # define I2C_100_KHZ // Comment out the following define statement to disable clock // stretching in i2cRecv () #define I2C_CLOCK_STRETCHING / * USER MUST CUSTOMIZE THE FOLLOWING DEFINE STM -END * / Note: The user-defined code is implemented at compile time, so the runtime is fixed.
Selecting SCL and SDA pins requires selecting two GPIO pins for SCL and SDA. After I / O is selected for SCL and SDA, the SDA_PORT and SCL_PORT definition statements must be edited to reflect the ports required by SDA and SCL. The SDA_PORT_BIT and SCL_PORT_BIT definition statements must also be edited to reflect the pins required by SDA and SCL (on the selected port).
The source code in Listing 1 above assigns pin 0 on I / O port 0 as SDA and pin 1 on I / O port 0 as SCL.
Select the communication rate by selecting one of the two definition statements I2C_400_KHZ and I2C_100_KHZ to select the communication rate.
The source code of Listing 1 initializes the maxqi2c library through the 400kHz I²C bus to communicate. Since the I²C interface is controlled bit by bit, the communication rate is actually slightly lower than 400kHz (or, alternatively, 100kHz). In order to achieve full-speed 400kHz communication, the firmware designer must learn the maxi2c library, remove some source code, and play the flexibility of the library.
Note: The maxqi2c library includes delays to meet I²C specifications. These delays are at the beginning of the maxqi2c.c file, assuming that the MAXQ2000 has a 20MHz system clock; if a lower rate clock is used, the delay can be reduced.
Using clock extension The clock extension of the maxqi2c library is only used when the transfer is started by calling the i2cRecv () function (address transfer is complete, after address confirmation, or at the start of transfer). Therefore, I²C transmission can use the clock extension in the following format: [S] [ADDR] [R] [A] [clock stretch] [DATA0] [A] ... [DATAN-1] [A] or [clock stretch] [DATA0] [A] ... [DATAN-1] [N] [P] or [clock stretch] [DATA0] [A] ... [DATAN-1] [A] Use i2cRecv (in maxqi2c section) ) Note that the code in the maxqi2c library usage examples section explains how to generate I²C commands in these formats.
To enable clock expansion, the I2C_CLOCK_STRETCHING definition statement should not be commented out. If clock expansion is not required, disable it by commenting out the I2C_CLOCK_STRETCHING definition statement. Disabling clock expansion will slightly increase the rate of the maxqi2c library i2cRecv () function.
The source code in Listing 1 above enables clock expansion. Using maxqi2c, the maxqi2c library sends and receives data from the software I²C driver by four functions: i2cInit (), i2cIsAddrPresent (), i2cSend () and i2cRecv (). The documentation for these functions is also included in the maxqi2c.h file.
These functions do not require formal parameters, but use 4 global variables to store parameters for these functions: i2cData (unsigned character *), i2cDataLen (unsigned integer), i2cDataAddr (unsigned character), and i2cDataTerm (unsigned character) . This method does not copy data when the function is called, thereby supporting the firmware to run at a faster rate. The four global variables used as parameters for the maxqi2c library are: i2cData (unsigned character *), i2cDataLen (unsigned integer), i2cDataAddr (unsigned character), and i2cDataTerm (unsigned character).
i2cInit () must call this function before calling any other maxqi2c function. It initializes the port pins selected by the user-defined code in the maxqi2c.h file. This function does not require parameters (local or global) and does not return values.
i2cIsAddrPresent () This function enables MAXQ2000 to query the I²C bus to determine if there is a device with a specific address. This function has one parameter-the global variable i2cDataAddr, which must be loaded by the device address, and query whether there is a device on the I²C bus. The function also returns a numeric value (unsigned character type). If a device with a given address is found, the value is equal to I2C_XMIT_OK, if no device with a given address is found, it is equal to I2C_XMIT_FAILED.
To determine whether there is a specific device on the I²C bus, i2cIsAddrPresent () sends an I²C command in the following format:
[S] [ADDR] [W] [A] [P] i2cSend () This function enables the MAXQ2000 to transfer data to the device through the software I2C driver. i2cSend () requires the following 4 parameters (all global variables) to initialize: i2cData (unsigned character *): the first byte pointer of the byte array to be transferred. i2cDataLen (unsigned integer): the number of bytes transferred to the I²C bus (excluding device address). i2cDataAddr (unsigned character): the address of the device to which the data will be transferred. Note that if this variable is set to 0, the address will not be sent and I²C data will be transferred. i2cDataTerm (unsigned character): how I²C transfer ends. When calling i2cSend (): I2C_TERM_NONE or I2C_TERM_STOP, this variable can take two values. The format for transferring data to the device on the I²C bus depends on the values ​​of 4 global variables. Table 1 lists the I²C command format when these global variables take different values.
Table 1. I²C commands sent by i2cSend ()
i2cDataLen (hex) | i2cDataAddr (hex) | i2cDataTerm | I²C Command Format |
0x0002 | 0x7E | I2C_TERM_STOP | [S] [ADDR] [W] [A] [DATA0] [A] [DATA1] [P] |
0x0002 | 0x7E | I2C_TERM_NONE | [S] [ADDR] [W] [A] [DATA0] [A] [DATA1] [A] |
0x0002 | 0x00 | I2C_TERM_NONE | [DATA0] [A] [DATA1] [A] |
0x0002 | 0x00 | I2C_TERM_STOP | [DATA0] [A] [DATA1] [A] [P] |
Note: The last three formats in Table 1 show how i2cSend () continuously sends data to the same device on the I²C bus.
If each byte of the addressed device responds, the i2cSend () function returns a value equal to I2C_XMIT_OK (unsigned character type). If the addressed device does not respond to each byte, the return value is equal to I2C_XMIT_FAILED. When a byte is not answered, the function will return immediately.
i2cRecv () This function enables the MAXQ2000 to use the software I²C driver to receive data from the device. The i2cRecv () function requires the following 4 parameters (all global variables) to initialize: i2cData (unsigned character *): stores the first byte pointer of the received data array. i2cDataLen (unsigned integer): the number of bytes received from the I²> C bus (excluding the device address). i2cDataAddr (unsigned character): the address of the device that will receive the data. Note that if this variable is set to 0, the address will not be sent and I²C data will be received. i2cDataTerm (unsigned character): how I²C transfer ends. When calling i2cRecv (): I2C_TERM_NONE, I2C_TERM_ACK or I2C_TERM_NACK_AND_STOP, this variable can take three values. The format of receiving data from the device on the I²C bus depends on the values ​​of 4 global variables. Table 2 lists the I²C command format when these global variables take different values.
Table 2. I²C commands sent by i2cRecv () with clock expansion disabled
i2cDataLen (hex) | i2cDataAddr (hex) | i2cDataTerm | I2C Command Format |
0x0002 | 0x7E | I2C_TERM_NACK_AND_STOP | [S] [ADDR] [R] [A] [DATA0] [A] [DATA1] [N] [P] |
0x0002 | 0x7E | I2C_TERM_ACK | [S] [ADDR] [R] [A] [DATA0] [A] [DATA1] [A] |
0x0002 | 0x00 | I2C_TERM_ACK | [DATA0] [A] [DATA1] [A] |
0x0002 | 0x00 | I2C_TERM_NACK_AND_STOP | [DATA0] [A] [DATA1] [N] [P] |
Note: The last three formats in Table 2 show how i2cRecv () continuously receives data from the same device on the I²C bus.
If the address is sent as part of an I²C command and no response is received, the i2cRecv () function returns a value (unsigned character) equal to I2C_XMIT_FAILED, otherwise, I2C_XMIT_OK is returned. Use example of maxqi2c library with clock extension The following example shows how to use the maxqi2c library to receive 16-bit samples from the MAX1169 ADC and transfer these data to the PC via the RS-232 port of MAXQ.
The principle is implemented using the MAX1169 ADC evaluation board and the MAXQ2000 evaluation board (Rev B). Figure 1 shows the connection of two evaluation boards. Pin 0 and pin 1 (J2-30 and J2-28, respectively) of the MAXQ2000 I / O port are used as the host SDA and SCL lines on the I2C bus.
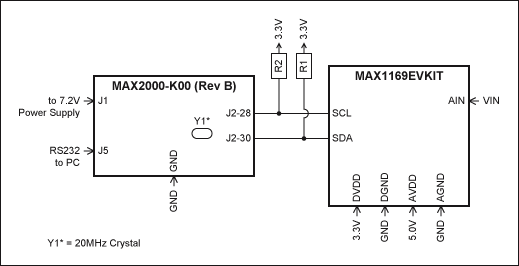
Figure 1. Schematic diagram of the connection between the MAX1169 evaluation board and the MAXQ2000 evaluation board (Rev B), which will be used by the maxqi2c library.
Note: The MAXQ2000 high-frequency crystal oscillator (Y1) on the MAXQ2000 evaluation board is replaced with a 20MHz crystal oscillator. The jumper settings of the MAX1169 evaluation board and the switch settings of the MAXQ2000 evaluation board are shown in Table 3 and Table 4:
Table 3: Jumper settings for the MAX1169 evaluation board
Jumper | Short circuit position |
JU1 | Install a shunt between pins 1 and 2 |
JU2 | Install a shunt between pins 1 and 2 |
JU3 | Install a shunt between pins 1 and 2 |
JU4 | No short circuit |
JU5 | No short circuit |
Table 4. MAXQ2000 Evaluation Board (Rev B) switch settings
switch | position |
SW1-1 | disconnect |
SW1-2 | disconnect |
SW1-3 | disconnect |
SW1-4 | turn on |
SW1-5 | disconnect |
SW1-6 | disconnect |
SW1-7 | turn on |
SW1-8 | disconnect |
SW6-1 | disconnect |
SW6-2 | disconnect |
SW6-3 | disconnect |
SW6-4 | disconnect |
SW6-5 | disconnect |
SW6-6 | disconnect |
SW6-7 | disconnect |
SW6-8 | turn on |
Firmware The firmware file for this example (max1169.c) is given in Appendix A. The complete engineering information can be downloaded from the Maxim MAXQ2000 web page and compiled using the MAXQ IAR embedded platform. In this example, the user-defined code of the maxqi2c library (at the beginning of the maxqi2c.h file) is exactly the same as the source code of Listing 1.
The max1169.c file includes two header files: iomaxq200x.h and maxqi2c.h. Note that the iomaxq200x.h file in the example will ignore the iomaxq200x.h file in the IAR embedded platform of the MAXQ include path. The iomaxq200x.h file defines each port pin required by the maxqi2c library. The maxqi2c.h file is included to support the firmware to call maxqi2c library functions.
The firmware is divided into 5 steps, which are marked in the max1169.c file (see Appendix A).
The first step is to initialize UART0 and perform asynchronous communication at 19200bps. Note that if the MAXQ2000 system clock is not 20MHz, the allocation of register PR0 must be modified to obtain the desired baud rate.
Step 2 calls the i2cInit () function to initialize the pins used by the MAXQ2000 I2C bus.
Step 3 Initialize the parameters and call the i2cRecv () function. After the parameters are initialized, I²C commands are transmitted in the following format: [S] [ADDR] [R] [A] [clock stretch] [DATA0] [A] [DATA1] [A (terminaTIon)] Step 4 Set the address parameters Is 0. Make the i2cRecv () function transfer the I²C command in the following format: [clock stretch] [DATA0] [A] [DATA1] [A (terminaTIon)] Step 5 is a cycle with an indeterminate period. This loop calls i2cRecv () (in the format defined in step 4), and receives a 16-bit sample from the MAX1169. The 16-bit sample is transmitted by UART0 (MSB first) to the PC. Since the matching parameter i2cDataTerm is always equal to I2C_TERM_ACK, the cycle period is uncertain, and the MAX1169 will not see the stop state.
Appendix A: max1169.c / * * DEMO of maxqi2c Software I²C Driver * (uses evkits for the MAX1169 and MAXQ2000) * * by: Paul Holden-MAXIM INTEGRATED PRODUCTS * * * DESC: Test program for the maxqi2c.c / maxqi2c. h I²C * driver for the MAXQ2000. The program reads * 16-bit samples from the MAX1169 (running in * conTInuous conversion mode) and transmits them * using the UART0 port. * * NOTE-THE FOLLOWING CODE ASSUMES THE MAXQ2000 HAS * A Fsysclk = 20MHz. * / #Include "iomaxq200x.h" #include "maxqi2c.h" void main () {unsigned char data [2]; // 1. Init UART0 PD7_bit.bit0 = 1; // Set TX0 pin as output SCON0 = 0x42; SMD0 = 0x02; PR0 = 0x07DD; // 19200bps // 2. Init bit-banged I²C port i2cInit (); // 3. Send iniTIal I²C request // [S] [ADDR + R] [A] [clock_stretch] [DATA0] [A] [DATA1] [A (termination)] i2cData = (unsigned char *) (& data); // cast needed! i2cDataAddr = 0x7E; i2cDataLen = 0x0002; i2cDataTerm = I2C_TERM_ACK; i2cRecv (); // 4. Init continuous conversion // [clock_stretch] [DATA0] [A] [DATA1] [A (termination)] i2cDataAddr = 0x00; // 5. Receive a 16-bit sample and transfer it to the UART0 port // one byte at a time. Repeat forever ... while (1) {i2cRecv (); while (! SCON0_bit.TI); // Wait for UART0 Buffer to be empty SCON0_bit.TI = 0; // Reset TI flag SBUF0 = data [0]; // Send data byte 0 while (! SCON0_bit.TI); // Wait for UART0 Buffer to be empty SCON0_bit.TI = 0; // reset TI flag SBUF0 = data [1]; // Send data byte 1}}
Indoor Fixed Advertising LED Billboard
This series is professionally used for indoor fixed installation of LED display, a variety of installation solutions such as wall installation, simple shelf installation, photo frame installation and so on. Displays advertising video content well indoors. It is suitable for bars, KTV, indoor meeting rooms, exclusive stores, inside shopping malls, indoor stores and so on. Indoor Fixed Advertising LED Billboard,beautiful cabinet design, seamless connection to realize large-screen display advertisement, high-definition smooth video playback function.
Indoor Fixed Advertising Led Billboard,Indoor Led Wall Billboards,Indoor Fixed Led Billboards,Shopping Mall Led Display
Guangzhou Cheng Wen Photoelectric Technology Co., Ltd. , https://www.leddisplaycw.com